Android SDK Integration
Introduction
Now with this Android SDK integration you can easily use Zaakpay in your android applications and can accept payments through various payment methods such as Credit and Debit Card, Net banking, UPI intent and UPI collect, Wallet etc for seamless flow and uninterrupted payments. Follow the steps carefully in order to have a bug free integration.
Have you generated the keys?
For the card transaction it is mandatory to generate the encryption keys. Please follow steps below to generate encryption and public keys:
- Login to the Zaakpay dashboard.
- Go to Configurations
- Click on API keys tab under that you'll find the Encryption Keys section. Kindly refer to the screenshot below for clear understanding.
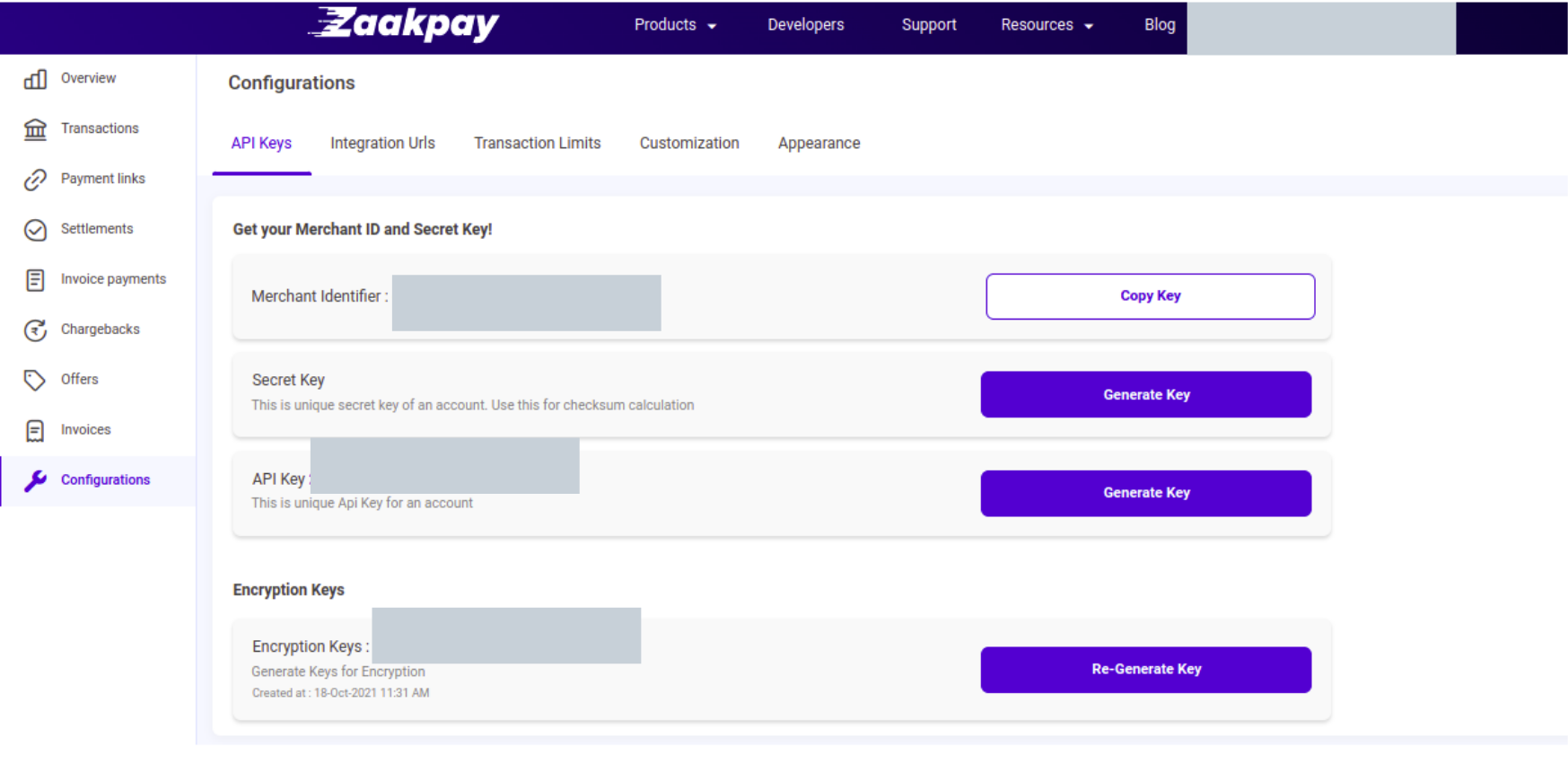
Want to check the payment flow?
Payment flow of Zaakpay LINK
Installing and Setting up SDK
Follow these steps in order to add all the dependencies that are required to setup Zaakpay payment gateway in your application.
Adding dependencies to your application
- Add the authToken for the repository access to your project’s gradle.properties file :
authToken=jp_nvgg9iebmirqko7uo25n0ssc9b
- Add the Jitpack repository url along with the authToken to your project level build.gradle file :
allprojects{
repositories{
maven {
url "https://jitpack.io"
credentials {
username authToken
}
}
}
}
}
- Add the SDK dependency to your app level build.gradle file :
implementation 'com.github.Mobikwik:Android-SDK:4.5'
Sync your gradle files after adding the dependencies and you should now be able to use the SDK in your application.
Latest Android SDK version
Android SDK version : 4.5
Initiating a payment through SDK
For initiating a payment through the SDK in your application, please undertake the following steps
- Create an instance of TransactionData by using TransactionDataBuilder
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/*****
application logic
****/
TransactionData transactionDataInstance = getTransactionDataInstance();
}
private TransactionData getTransactionDataInstance() {
TransactionDataBuilder transactionDataBuilder = TransactionDataBuilder()
.withAmount(amount) // BigInteger
.withChecksum(checksum) // String
.withChecksumPO(checksumPO) // String
.withCurrency("INR") // String
.withOrderId(orderId) // String
.withUserEmail(userEmail) // String
.withReturnUrl(returnUrl) // Url on which the txn response will be posted (String)
.withMerchantIconUrl(iconUrl) // Merchant Icon to be displayed in the SDK (String)
.withMerchantName(merchantName) // String
.withMerchantId(merchantIdentifier) // String
.withUserPhoneNumber() //String
.withEnvironment(Enums.Environment.STAGING); // Enum to configure the server environment
return transactionDataBuilder.build();
}
- The TransactionData object created above should then be passed in the startPayment of the PaymentCheckout class
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/*****
application logic
*****/
TransactionData transactionDataInstance = getTransactionDataInstance();
PaymentCheckout paymentCheckout = new PaymentCheckout(this);
paymentCheckout.startPayment(transactionDataInstance);
}
This will trigger the SDK flow inside your application and present a list of payment options to the user.
Note
In case you face Manifest merger failed : Attribute application@theme error while building your application with the SDK, please add tools:replace="android:theme" in the application tag inside your application’s AndroidManifest.xml file.
Checksum Calculation
The checksum and checksumPO values used here must be calculated and fetched from the merchant’s server.
The above example shows the mandatory params needed for the SDK transaction. You can check the full list of params supported by the TransactionData object here : LINK
For both integrity & data-authenticity verification before sending data to the API. We use an algorithm HMAC SHA-256 to calculate the checksum.
There are two checksums that are created in the process of transaction i:e Checksum PO and Checksum
ChecksumPO Calculation
Checksum PO string consists of merchantIdentifier and emailId. String should be created in the format as given below.
Considering staging Credentials and email id as '[email protected]' :
- Merchant Identifier : b19e8f103bce406cbd3476431b6b7973
- Secret key : 0678056d96914a8583fb518caf42828a
merchantIdentifier=b19e8f103bce406cbd3476431b6b7973&[email protected]
f9ea7ead1b43f028795eed9fdedd0721b00dd660999242eee0ff10560bac4602
Checksum Calculation
Checksum string consists of parameters in the same order merchant Identifier, orderid, mode, currency, amount, Buyer's Device IP, date
Considering staging Credentials and email id as '[email protected]' :
- Merchant Identifier : b19e8f103bce406cbd3476431b6b7973
- Secret key : 0678056d96914a8583fb518caf42828a
'b19e8f103bce406cbd3476431b6b7973''1551264623988gjgkgf''0''INR''200''ip''date'
Register Callback Listener
Post the completion of the payment process we need to get a final transaction response from the SDK. In order to do that we will be using Register Callback Listener, you must implement the PaymentCheckout.ZaakPayPaymentListener inside your activity/fragment from which you initiated the payment.
public class PaymentActivity extends AppCompatActivity implements PaymentCheckout.ZaakPayPaymentListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
/* application checkout flow logic */
TransactionData transactionDataInstance = getTransactionDataInstance();
PaymentCheckout paymentCheckout = new PaymentCheckout(this);
paymentCheckout.startPayment(transactionDataInstance);
}
@Override
public void onPaymentSuccess(TransactionResponse response) {
// extract the checksum from the response using response.getChecksum() and verify it on your server before further processing the success response
}
@Override
public void onPaymentFailure(String responseCode, String responseDescription) {
//handle payment failure
}
}
Transaction Flow
After completing all the above steps you are good to go and test your integration. Below is a demo application demonstrating the Zaakpay payment integration in android.
Want to test our demo application?
Link to the application : LINK
To verify the authenticity of the response received in case of payment success it is recommended to extract the checksum from the response and verify it on your server before any further processing. This helps fight against MITM attacks or network fiddling. The specifics on verifying the response checksum on the merchant server is available in next section.
Following sample response should be returned in response of return url. You will get the values of these parameters in the request parameters of return url hit.
Return/Redirect URL
The return URL is the URL on which the response of the transaction will be posted. On completion of transaction Zaakpay will redirect the user to the merchant's platform.
Sample Response:
{
"orderId": "1601294679223",
"responseCode": "100",
"responseDescription": "The transaction was completed successfully. ",
"checksum": "1b6f38fca8ceb319218d806f79f7e163de595eb8b3753c976441733028f186b8",
"amount": "100",
"doRedirect": "false",
"paymentMode": "UPI",
"cardId": "NA",
"cardScheme": "NA",
"cardToken": "NA",
"bank": "ICICIUPI",
"bankid": "ICIUPI",
"paymentMethod": "N1064",
"cardhashid": "NA",
"product1Description": "NA",
"product2Description": "NA",
"product3Description": "NA",
"product4Description": "NA",
"pgTransId": "ZP5b05e7c3666b8",
"pgTransTime": "09/28/2020 17:35:54"
}
Response Checksum
If you want to validate the checksum on the above JSON response string. Please follow below required setps:
- Use SHA -256 algorithm to generate the correct checksum for JSON string
- We need to calculate on all the parameters of the JSON string
- Use merchant's secret key for the same
Sample Input Parameters:
amount=100&bank=ICICIUPI&bankid=ICIUPI&cardId=NA&cardScheme=NA&cardToken=NA&cardhashid=NA&doRedirect=false&orderId=1601294679223&paymentMethod=N1064&paymentMode=UPI&responseCode=100&responseDescription=The transaction was completed successfully. &product1Description=NA&product2Description=NA&product3Description=NA&product4Description=NA&pgTransId=ZP5b05e7c3666b8&pgTransTime=09/28/2020 17:35:54&is 1b6f38fca8ceb319218d806f79f7e163de595eb8b3753c976441733028f186b8
Secret Key: Please user merchant's secret Key
Zaakpay Payment Gateway Response codes
These are the transact API response codes. For more response code, Please download Document Directly from here.
Updated over 2 years ago